What are transactions in C#? (.NET interview questions with answers)
- By Shiv Prasad Koirala in .Net
- Sep 19th, 2013
- 14976
- 0
Transaction is a set of operations that should be considered as one unit.
Either every operations will executes successfully or none will. It should not end up in middle.
Best Example for transaction is money transactions in banks where X amount will be transferred from Account A to Account B. Steps are
1. Deduct Amount X from Account A
2. Add Amount X to Account B.
Let say after step 1 and before step 2 power failure happen. In that case step 1 will also get rolled back because both the steps together are considered as one Transaction
So how to do we implement a transaction in .NET?
To implement transactions we can use the transaction scope object as shown in the below code.
using(var scope = new TransactionScope())
// Do all your insert,update and deletes here
scope.Complete(); //if we make it here - commit the changes,
//if not - everything is rolled back
}
How do we implement transactions in SQL Server stored procedure?
To implement transaction in SQL Server we need use "Begin transaction" and "Commit" transaction as shown in the below code.
BEGIN TRANSACTION [Tran1]
BEGIN TRY
INSERT INTO [Test].[dbo].[T1]
([Title], [AVG])
VALUES ('xyz', 130), ('pqr', 230)
UPDATE [Test].[dbo].[T1]
SET [Title] = N'abc' ,[AVG] = 1
WHERE [dbo].[T1].[Title] = N'az'
COMMIT TRANSACTION [Tran1]
END TRY
BEGIN CATCH
ROLLBACK TRANSACTION [Tran1]
END CATCH
How do we implement transaction using ADO.NET ?
Connection object of ADO.NET has a transaction object we can use that.
SqlConnection db = new SqlConnection("connstringhere");
SqlTransaction transaction;
db.Open();
transaction = db.BeginTransaction();
try
new SqlCommand("INSERT INTO TransactionDemo " +
"(Text) VALUES ('Row1');", db, transaction)
.ExecuteNonQuery();
new SqlCommand("INSERT INTO TransactionDemo " +
"(Text) VALUES ('Row2');", db, transaction)
.ExecuteNonQuery();
new SqlCommand("INSERT INTO CrashMeNow VALUES " +
"('Die', 'Die', 'Die');", db, transaction)
.ExecuteNonQuery();
transaction.Commit();
}
catch (SqlException sqlError)
transaction.Rollback();
}
db.Close();
Do miss the below SQL Server interview question video :- Can views be updated ?
Shiv Prasad Koirala
Visit us @ www.questpond.com or call us at 022-66752917... read more
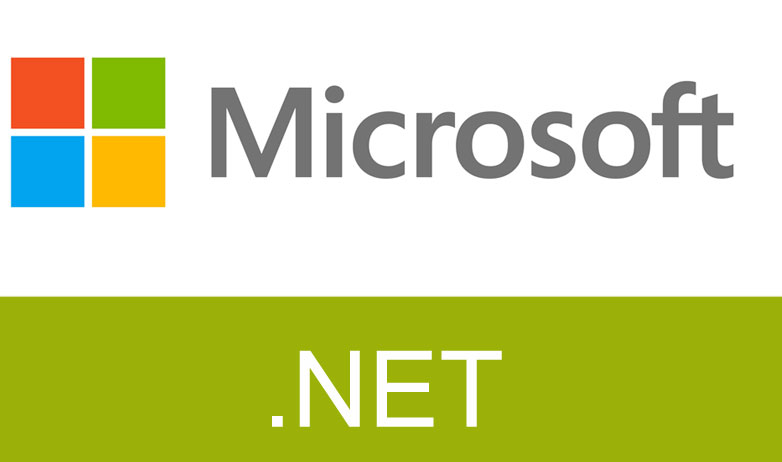
- By Shiv Prasad Koirala
- Jun 21st, 2013
- 162189
- 0
.NET interview questions 6th edition (Sixth edition) - By Shivprasad Koirala
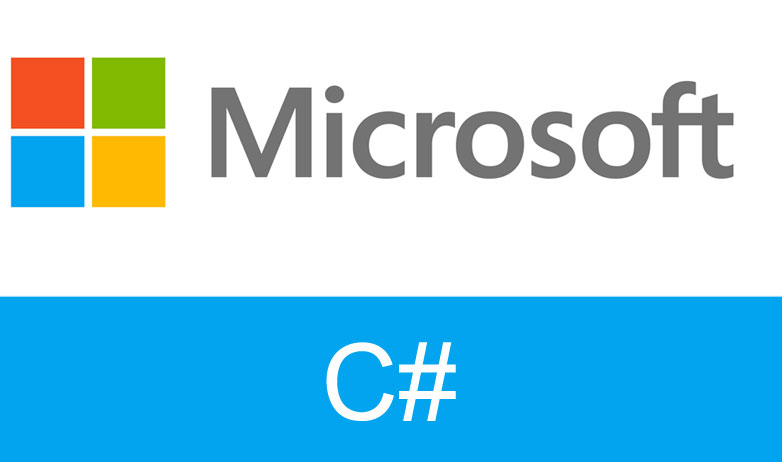
- By Shiv Prasad Koirala
- Dec 8th, 2016
- 88136
- 0
Exception Handling in C# using example step by step
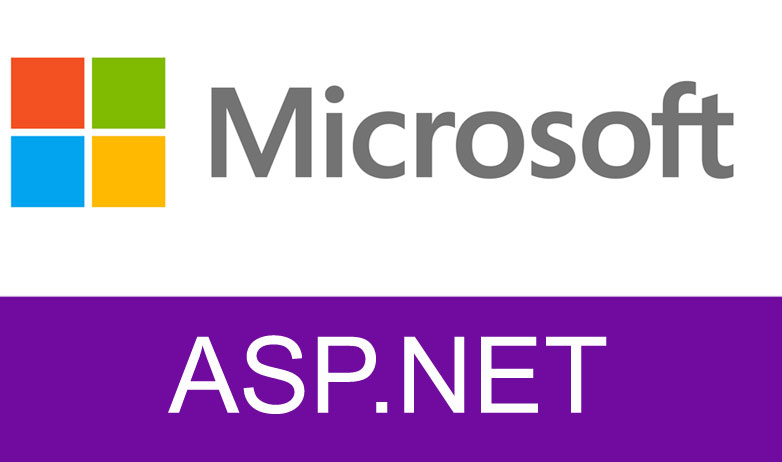
- By Shiv Prasad Koirala
- Sep 7th, 2013
- 71590
- 0