C# Constructor Interview Questions
- By Shiv Prasad Koirala in C#
- Sep 12th, 2016
- 19112
- 0
In the interview when basics of C# topic come, Constructor is one of the sub topics which are asked frequently. So let us discuss some questions about Constructor.
What is the use of Constructor?
To answer this question first we will know what a constructor is in C #?
Whenever a class or a struct (structure) is created, its constructor is called. They have the same name as the class or struct. The use of Constructor is to enable the programmers to set default value, write code that is flexible and easy to read. Usually they initialize the data members of a new object.
In the Child class can the Parent class constructor be inherited?
Now basically in a parent child relationship a child class can inherit all the methods and functions from the parent class. So in the same way if a parent class is having a constructor and a child class wants to use it, it can definitely inherit the constructor from the parent class. Here we have to use the "base"keyword for this kind of initialization.
public class mathclass public mathclass() // This is the constructor method. } // rest of the class members goes here. } mathclass obj = new mathclass() // At this time the code in the constructor will // be executed
This is how the simple constructor looks like. So basically constructor is a method in the class which gets executed when an object is created. We put the initialization code inside the constructor. Also how the "base" key word is used is shown here,
public class mathclass public mathclass() // This is the constructor method. } // rest of the class members goes here. } public class advancemathclass : mathClass public advancemathclass():base() // Code for the advancemathclass Constructor. } // Other class members goes here }
Here we saw how the "base" keyword is used to call the constructor from the parent class in our child class.
What is the sequence of firing of the Constructors?
As we saw in the above example how "base" key word is used for inheriting the constructor from the parent class to child class. So now the firing sequence or the execution sequence will be very simple,
- First the mathclass() method will be executed and then
- The advancemathclass() method will be executed.
Now suppose if we are having a Constructor with some parameter and a Constructor with no parameter in both parent and child classes, then the execution sequence will be first for the parent class constructor with no parameter and then the child class constructor with no parameter. Then for constructors with some parameters the firing sequence is same as above, first the parent class constructor and then the child class constructor.
Also when we are writing the "base" keyword, it represents the base class or the parent class. So here also when "base" keyword is written
- First the base class or parent class Constructor is fired and then,
- The child class constructor is fired.
If we do not mention the "base" keyword, then by default it follows the above execution sequence.
How to call in child class the base class Constructor?
Now the question is same like the previous one where we discussed how the child class can inherit the parent class Constructor.
So the answer to this question is that child class can inherit from the base class or parent class Constructor. And to call in the base class Constructor inside the child class we use the "base"keyword. We saw the above example where we have used "base" keyword.
Also watch our latest video on C# interview questions & answers :-
Shiv Prasad Koirala
Visit us @ www.questpond.com or call us at 022-66752917... read more
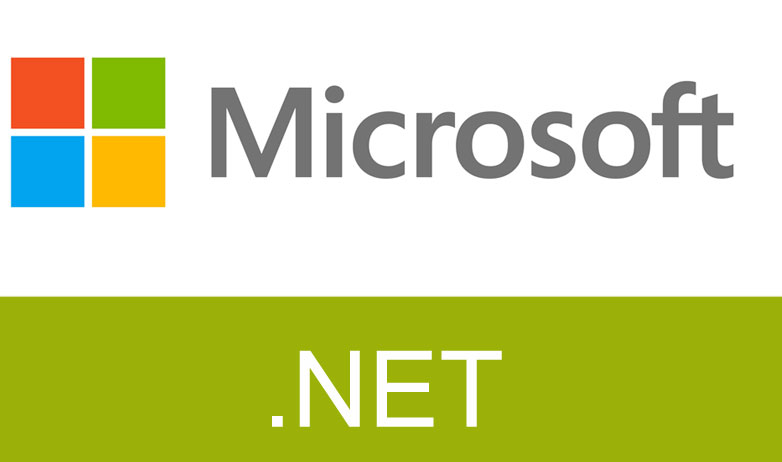
- By Shiv Prasad Koirala
- Jun 21st, 2013
- 165277
- 0
.NET interview questions 6th edition (Sixth edition) - By Shivprasad Koirala
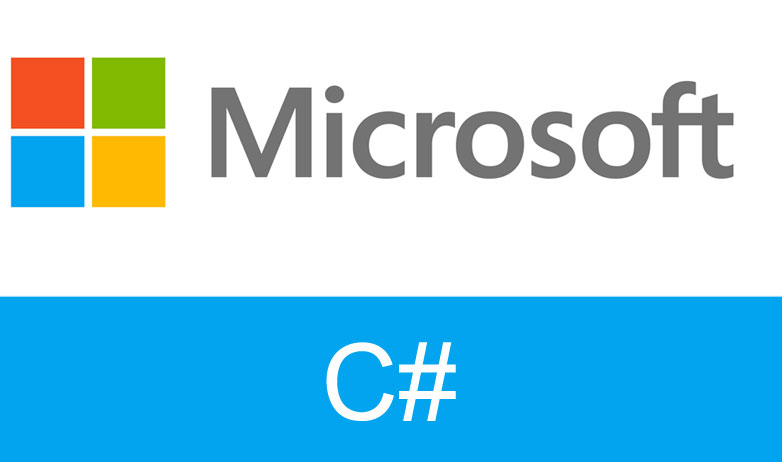
- By Shiv Prasad Koirala
- Dec 8th, 2016
- 89490
- 0
Exception Handling in C# using example step by step
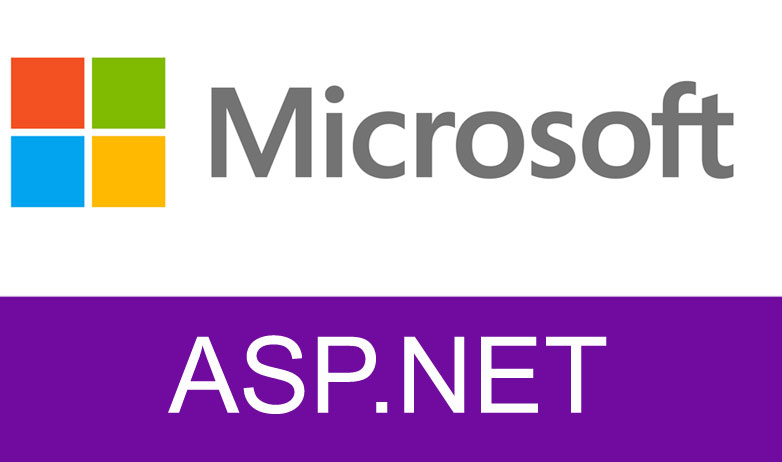
- By Shiv Prasad Koirala
- Sep 7th, 2013
- 72282
- 0