Explain Stack, Heap, Value type & reference type in C#
- By Shiv Prasad Koirala in C#
- Dec 23rd, 2022
- 3288
- 0
Introduction to Stack and Heap in C#
Stack and Heap are memory types where application running variables, object variables, objects references are stored. Both are stored in RAM. Normally when we executes the program certain amount of memory is required to run or compile the program so in our C# application memory allocation can be done either in stack or heap according to the type and condition of the variable.
Generally our stand alone primitive data types are stored on stack and object and and string datatypes are stored in heap. For knowing both in depth lets we discuss it in separate:
Stack
- The memory which is used to store and manage primitive data types, object references pointers on the execution of the of the program is known as stack memory in C#.
- Stack memory is contiguous and memory address do not have gaps.
- Elements are added and removed means memory deallocation in LIFO(Last In First Out)manner.
- In stack memory addresses are directly points outs the value and in case of objects pointers they are not able points out the actual data but they points outs the memory addresses.
- Note this point only stand alone primitive datatypes and object pointer will stored in stack.
- It is faster but it has limited size for memory so if objects are created more there is an chances of stack overflow.
- stack overflow: if the system creates more objects then that of its size which results in failure of the program then that condition is called stack overflow.
Heap
This is all about stack and heap memory now we go through simple example then we are clear about it.
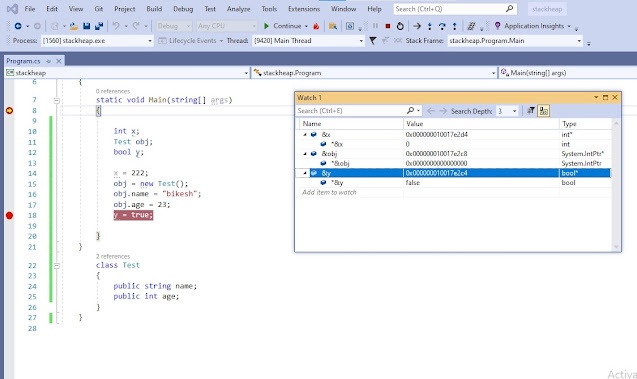
In the above screen we write a simple program having different variables like x as integer , y as Boolean and test obj as object which also contains different variables like name as string and age as integer. Now when we run the program with debug points then it clearly show stand alone primitive datatypes means integer and Boolean data type are directly stores their value inside it but object should hold some memory address inside it which address is totally different from other memory addresses.
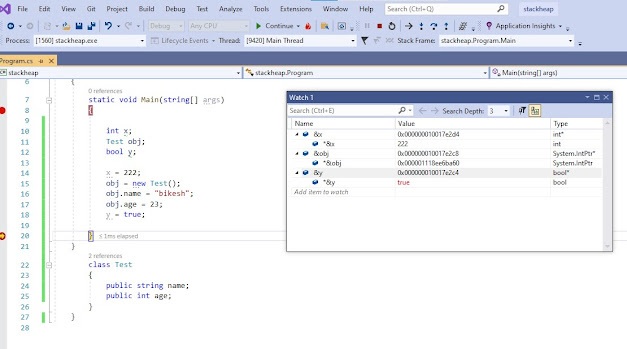
from above we have an clear idea about what type of variables are stored in heap and what type of variables stored in stack. normally those stand alone primitive datatypes and object pointers are stored in stack and actual object and string datatypes are stored in heap. Objects pointers can holds their memory location in stack.
Value types and Reference Types in C#:
Generally value types means primitive data types and reference types means an objects. Value types contains the data inside its own memory allocation while in reference types the pointer should be stored in one memory allocation i.e. in stack and their value or data should be stored in other memory allocation i.e. in heap.
- When you assign new one integer to an other integer it should create a new fresh memory address for that new integer. While in reference types both variables have different memory allocations but both can share common memory address.
- We can say value types are primitive types vary in the value through value are copied and fresh memory is created. Simply copy by values means Value type and copy by memory addresses means reference type.
- We can say reference type are actually moves the object reference and copied the object reference. they point out towards the same data if it will changed for one places then it should be changed for every objects. Let we discuss it through an example:
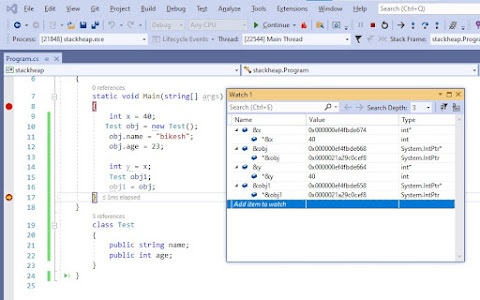
In the above example we write a simple program that has both primitive and objects types where we are trying to assign one integer to another integer and one object to another objects then we see integer datatypes x and y can copy by the value 40 having different memory address for different variable. But in obj and obj1 both object pointer have different memory addresses but both can hold same memory address /0x0000021a29c0cef8/ from that it is clear value type creates new memory addresses for new variable but in reference type different objects pointers having different memory addresses can share common memory addresses.
1 Hour detailed video on Stack VS Heap VS Value Type VS Reference Type:-
Shiv Prasad Koirala
Visit us @ www.questpond.com or call us at 022-66752917... read more
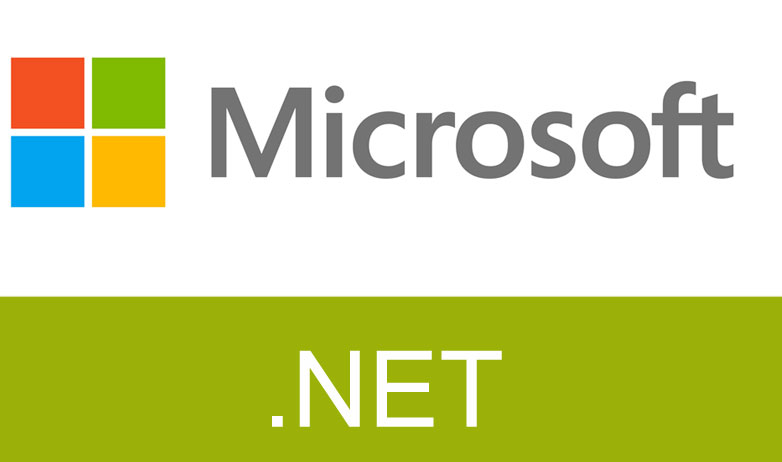
- By Shiv Prasad Koirala
- Jun 21st, 2013
- 165265
- 0
.NET interview questions 6th edition (Sixth edition) - By Shivprasad Koirala
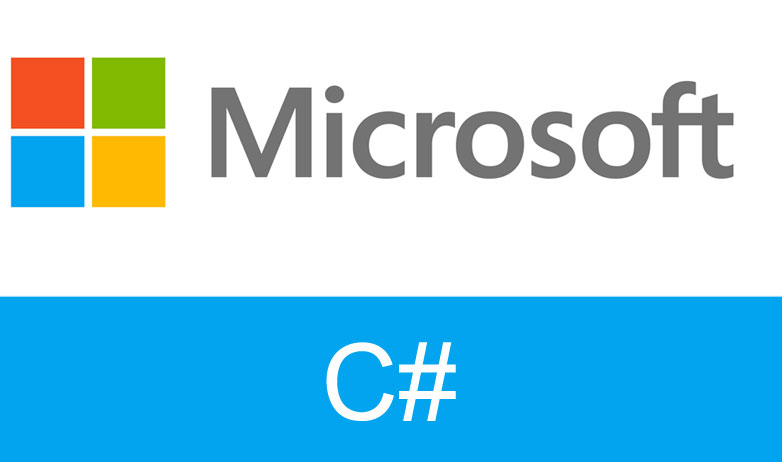
- By Shiv Prasad Koirala
- Dec 8th, 2016
- 89488
- 0
Exception Handling in C# using example step by step
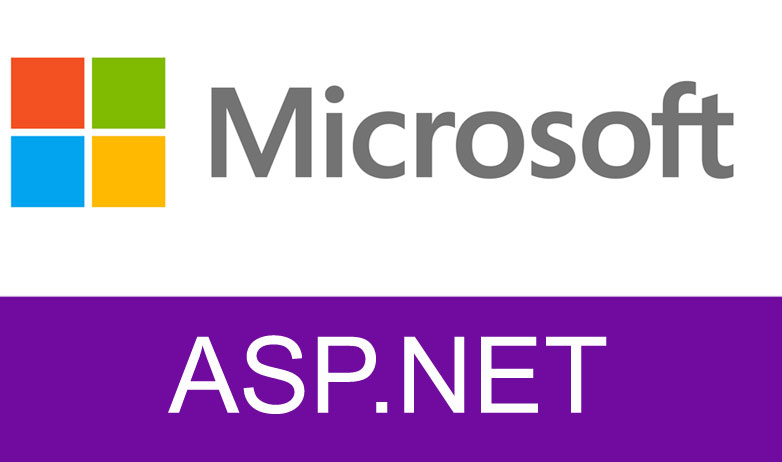
- By Shiv Prasad Koirala
- Sep 7th, 2013
- 72280
- 0