Serialization and Deserialization in C# step by step
- By Shiv Prasad Koirala in C#
- Dec 9th, 2016
- 49632
- 0
Serialization
Serialization is converting object to stream of bytes. It is a process of bringing an object into a form that it can be written on stream. It's the process of converting the object into a form so that it can be stored on a file, database or memory. It is transferred across the network. Main purpose is to save the state of the object.
First, we go and import the name space for serialization.
usingSystem.Runtime.Serialization;
Then Import the formatter
using System.Runtime.Serialization.Formatters.Binary;
Convert "customer obj" to binary. Also, need the "using System.IO;"create the file or read to file. Most important, go and mark the "class".
Advantages of serialization
- Passing an object from one application to another.
- Passing an object from one domain to another.
For example:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Runtime.Serialization;
using System.Runtime.Serialization.Formatters.Binary;
using System.IO;
namespace serialization
classProgram
staticvoid Main(string[] args)
//SERIALIZATION
//step 1
customer objcust = newcustomer();
objcust.CustomerName = "RAHUL";
objcust.CAddress= "VARANASI";
// object create for the binary formatter to save to file
IFormatter ibin = newBinaryFormatter();// create the binary formatter
Stream strobj = newFileStream(@"f:\MyFile.obj", //file location
FileMode.Create, // create folder
FileAccess.Write, // write the file
FileShare.None);
ibin.Serialize(strobj, objcust); //written to the file
strobj.Close();
}
}
[Serializable] // also go and mark the class
classcustomer
privatestring _CustomerName;
publicstring CustomerName
get return _CustomerName; }
set _CustomerName = value; }
}
privatestring _CAddress;
publicstringCAddress
get return _CAddress; }
set _CAddress= value; }
}
}
}
File in created to given the drive.
Deserialization
Deserialization is converting stream of byte to object. Deserialization is the reverse process of serialization. It is the process of getting back the serialization object (so that) it can be loaded into memory. It lives the state of the object by setting properties, fields etc.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Runtime.Serialization;
using System.Runtime.Serialization.Formatters.Binary;
using System.IO;
namespace serialization
classProgram
staticvoid Main(string[] args)
//SERIALIZATION
//step 1
customer objcust = newcustomer();
objcust.CustomerName = "RAHUL";
objcust.CAdd = "VARANASI";
// object create for the binary formatter to save ti file
IFormatter ibin = newBinaryFormatter();// create the binary formatter
Stream strobj = newFileStream(@"f:\customer.obj", //file location
FileMode.Create, // create folder
FileAccess.Write, // write the file
FileShare.None);
ibin.Serialize(strobj, objcust); //written to the file
strobj.Close();
//DESERIALIZATION
customer objdeseri = newcustomer();
// object create for the binary formatter to save ti file
IFormatter ibindeseri = newBinaryFormatter();// create the binary formatter
Stream strobjdeseri = newFileStream(@"f:\MyFile.obj", //file location
FileMode.Open, // open the file
FileAccess.Read, // read the file
FileShare.None);
objdeseri = (customer)ibindeseri.Deserialize(strobjdeseri); //written to
the file
strobjdeseri.Close();
}
}
[Serializable] // also go and mark the class
classcustomer
privatestring _CustomerName;
publicstring CustomerName
get return _CustomerName; }
set _CustomerName = value; }
}
privatestring _CAddress;
publicstringCAddress
get return _CAddress; }
set _CAddress = value; }
}
}
}
Reverse the data from the file.
Also get to see following video on Learn C# tutorial for beginners: -
Shiv Prasad Koirala
Visit us @ www.questpond.com or call us at 022-66752917... read more
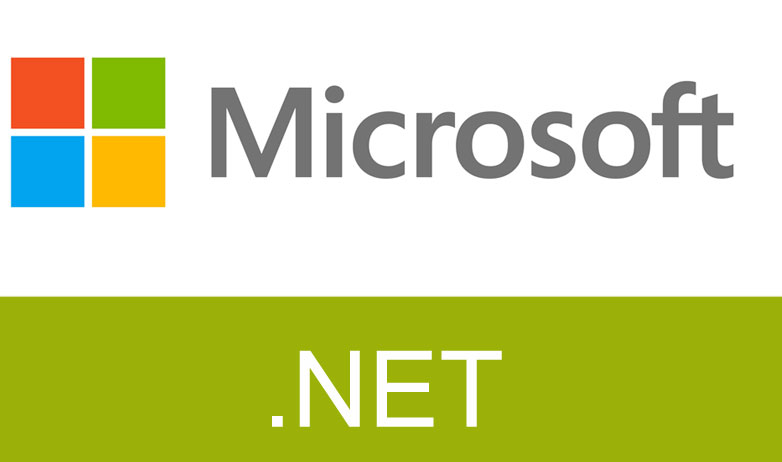
- By Shiv Prasad Koirala
- Jun 21st, 2013
- 162684
- 0
.NET interview questions 6th edition (Sixth edition) - By Shivprasad Koirala
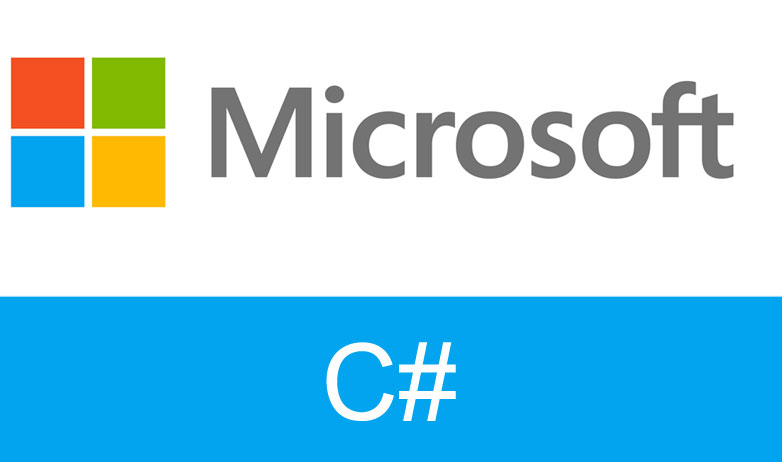
- By Shiv Prasad Koirala
- Dec 8th, 2016
- 88608
- 0
Exception Handling in C# using example step by step
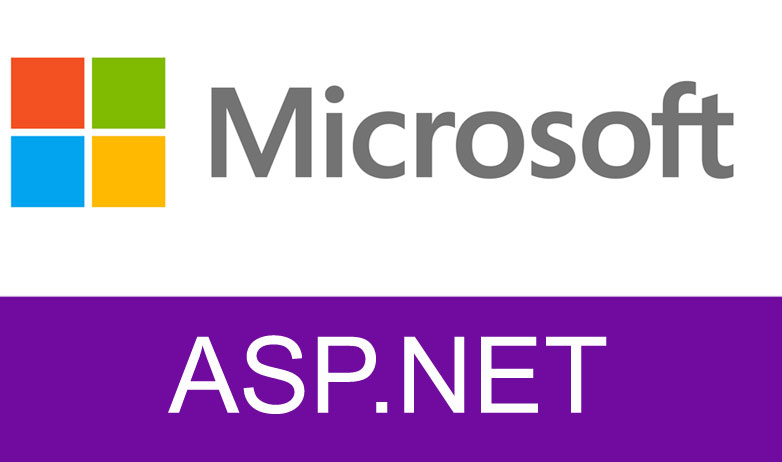
- By Shiv Prasad Koirala
- Sep 7th, 2013
- 71694
- 0