What is the difference between explicit and implicit interface implementation (C# interface interview questions)?
- By Shiv Prasad Koirala in C#
- Oct 27th, 2013
- 11289
- 0
Sometimes this question is twisted and asked in a different way "Can we implement interfaces with same method names in c# ?".
As all of us know one of the advantage of using interfaces is to support multiple inheritance where one class will implement more than one interface.
Example:
public interface I1
void method1();
}
public interface I2
void method2();
}
public class MyClass:I1,I2
public void method1()
//do something here
}
public void method2()
//do something here
}
}
.
.
I1 i=new MyClass();
i.method1(); //method2 will not be available.
I2 i2=new MyClass();
i2.method2(); //method1 will not be available.
These kind of implementation is called as implicit interface implementation.
Now let's say both of these interface have a method called Method3, Now the question is how they will be defined in MyClass.
public class MyClass:I1,I2
public void method1() // Because of I1
//do something here
}
public void method2() //Because of I2
//do something here
}
public void method3() //Because of both I1 and I2
//do Something here
}
}
In above code we are using Implicit implementation so both of the following call will do same thing.
I1 i=new MyClass();
i.method3();
I2 i2=new MyClass();
i2.method3();
Now what if we want to write separate implementation for both the interface methods. There comes explicit interface implementation.
public class MyClass:I1,I2
public void method1() // Because of I1
//do something here
}
public void method2() //Because of I2
//do something here
}
public void I1.method3() // Because of I1
//do something here
}
public void I2.method3() // Because of I2
//do something here
}
}
A big thanks to questpond.com to provide this C# interface interview question.
Also watch our latest video on C# interview questions & answers :-
Shiv Prasad Koirala
Visit us @ www.questpond.com or call us at 022-66752917... read more
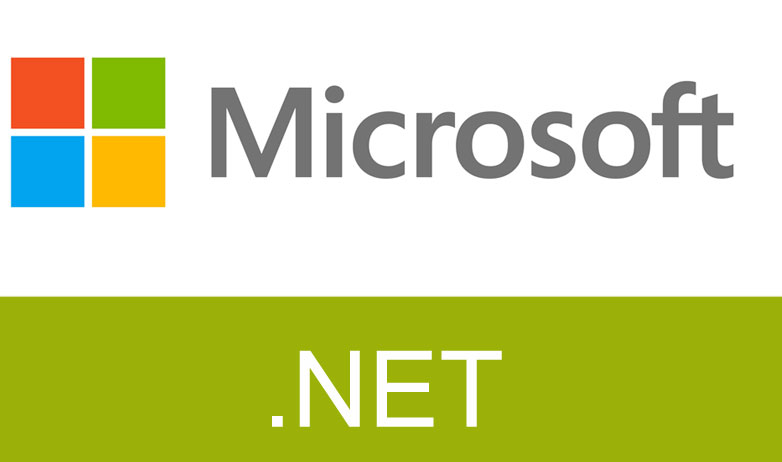
- By Shiv Prasad Koirala
- Jun 21st, 2013
- 165843
- 0
.NET interview questions 6th edition (Sixth edition) - By Shivprasad Koirala
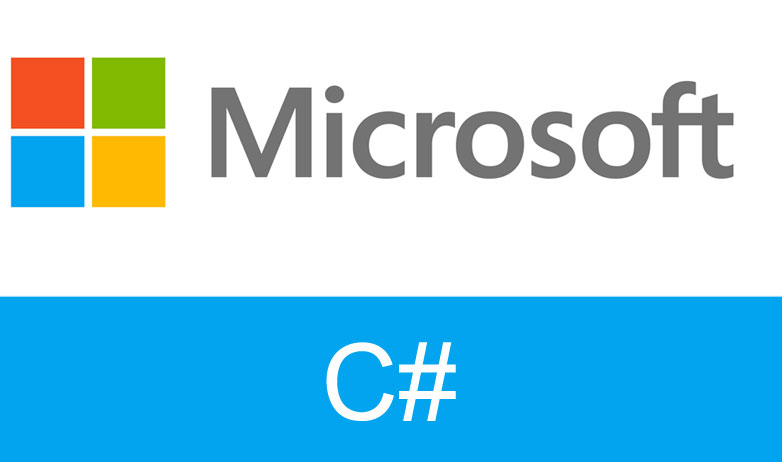
- By Shiv Prasad Koirala
- Dec 8th, 2016
- 89606
- 0
Exception Handling in C# using example step by step
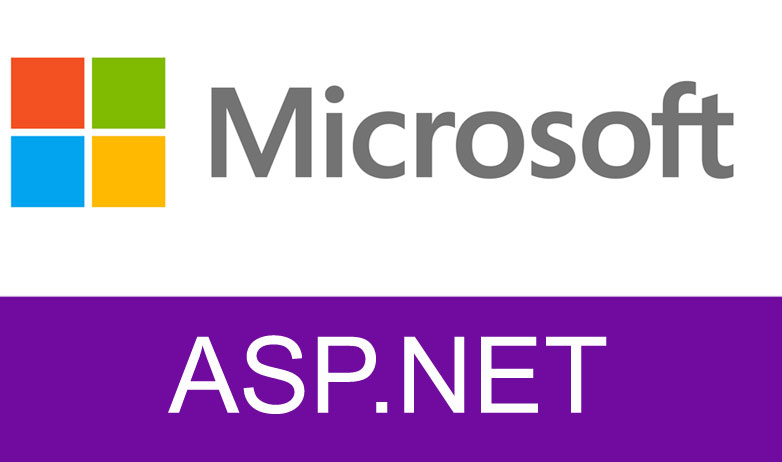
- By Shiv Prasad Koirala
- Sep 7th, 2013
- 72355
- 0